There’s a growing demand to create more polished user experiences in increasingly complex web applications. Making the jobs of QA engineers more important - and more difficult - than ever.
The ability to simulate and mock data and APIs effectively is crucial for efficient testing and development. Luckily, there are some great tools to make this process much smoother: Cypress and Mock Service Worker (MSW).
Table of Contents |
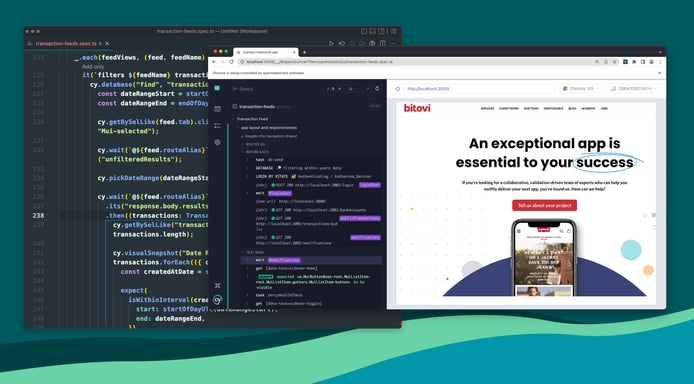
What is Cypress?
Cypress is an open source, end-to-end testing framework that operates directly in the browser. The browser-based framework makes testing more observable and allows testers to control state and test in real-time. Cypress is designed to simplify testing for even the most complex applications and offers a fast and reliable way to test an application's functionality. It’s also tech stack agnostic, meaning once you master all of Cypress’ capabilities, you can use it to test anything that runs in a browser!
What is MSW?
MSW, short for Mock Service Worker, is a library for intercepting and mocking HTTP requests in the browser. It enables you to simulate server responses without making actual network calls. MSW can be used independently in any JavaScript environment, but it also works seamlessly with Cypress to provide a comprehensive mocking solution.
Why Mocking Matters
Mocking data and APIs is essential to maximizing the efficiency of a product cycle. Whether it is best practice or not, testing can often happen towards the end of the product development cycle—sometimes just before the application goes to market, leaving a very small window for recoding and retesting. Insufficient testing windows can lead to a product’s diminished business value and frustration for developers and stakeholders alike.
To maximize that small testing window, it’s helpful to decouple your frontend from backend services so each part can be worked on each part independently. This cuts down on back and forth between teams and means coding and testing can be done in isolation.
Speed also plays a role. Simulated responses are faster than actual network requests, leading to quicker test execution and development feedback loops.
Of course, all of that isn’t worth it if your testing isn’t reliable. Testing against known mock data ensures consistent results, reducing the likelihood of false positives or negatives in your tests.
Setting Up Cypress and MSW
Prerequisites
Node.js: Ensure you have Node.js installed on your machine. MSW and Cypress require Node.js to run.
Installation Steps
-
Create a new Node.js project (if needed):
If you haven't already set up a Node.js project, create a new directory for your project and initialize a new Node.js project using npm or yarn.
mkdir your_project_directory cd your_project_directory npm init -y
-
Install Cypress:
Install Cypress as a development dependency in your project using npm or yarn.
npm install cypress --save-dev
-
Install Mock Service Worker (MSW):
Install MSW and other necessary dependencies.
npm install msw --save-dev
-
Create a MSW setup file:
In your project, create a file named
msw.setup.js
(or any other name you prefer). This file will configure MSW for your Cypress tests.// msw.setup.js import { setupWorker } from 'msw'; import { handlers } from './handlers'; // Create this file for your API handlers const worker = setupWorker(...handlers); worker.start();
-
Create API handlers:
Create a file named
handlers.js
to define your API handlers using MSW.// handlers.js import { rest } from 'msw'; export const handlers = [ rest.get('/api/endpoint', (req, res, ctx) => { return res(ctx.json({ message: 'Mocked response' })); }), ];
-
Modify Cypress plugins file:
Open
cypress/plugins/index.js
and import the MSW setup file. This will ensure MSW is started before your Cypress tests.// cypress/plugins/index.js import '../../msw.setup.js'; // Rest of your existing plugins setup...
-
Update Cypress configuration:
Open
cypress.json
and add the following configuration to ensure Cypress loads the plugins and sets up the base URL.{ "baseUrl": "<http://localhost:3000",> "pluginsFile": "cypress/plugins/index.js" }
-
Write your tests:
Write your own tests using Cypress. Tests are typically written in JavaScript using Cypress's API.
-
Navigate to the
cypress/integration
folder and create a new test file (e.g.,example_spec.js
). -
Write your test cases using Cypress commands and assertions.
-
-
Run your tests:
Use the Cypress Test Runner or run Cypress in headless mode via the command line.
-
To run tests in the Test Runner:
npx cypress open
-
To run tests in headless mode (for CI/CD):
npx cypress run
-
For more advanced MSW usage and customization, be sure to check out their documentation.
Utilizing Mocks in Cypress Tests
With your mocks set up, you can now use them in your Cypress tests:
// cypress/integration/test.spec.js
describe('API Mocking Test', () => {
it('should mock a successful API response', () => {
cy.visit('/path-to-your-page')
// Assuming that page triggered a request to '/api/endpoint'
// MSW intercepts the request and responds with the mocked response
// Page loads with mocked data
cy.get('[data-testid="api-trigger"]').click()
cy.wait('@mockedResponse')
// Add assertions here
})
})
That’s a Wrap
Cypress and MSW provide a synergistic combination for simplifying data and API mocking in your development and testing workflows. With these tools, you can isolate frontend and backend development, speed up test execution, and enhance the reliability of your tests.
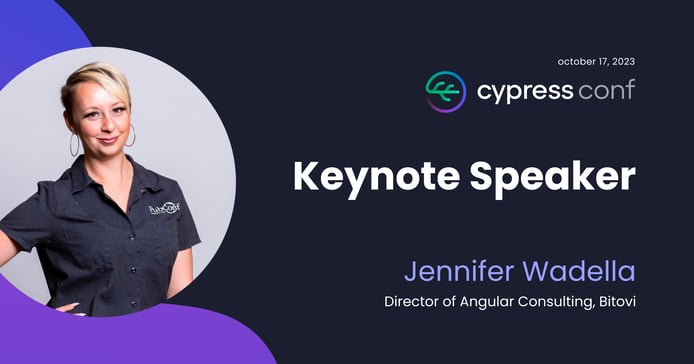
Are you looking to modernize your testing practices?
Join Bitovi’s Director of Angular, Jennifer Wadella, on October 17th as she delivers the keynote talk at Cypress Conference 2023. With the advent of advanced testing tools, there's immense potential for improved test infrastructure. However, many struggle with the testing-engineering divide. Jennifer's insights will help break down silos and promote a cohesive testing culture, using tools like Cypress to empower teams, prioritize testing, and enhance developer experiences.
Previous Post